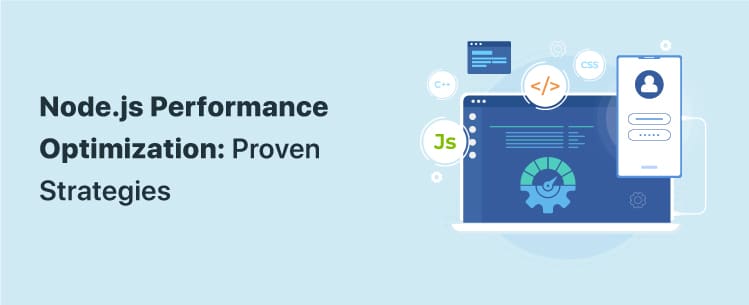
Quick Summary:
Delivering high-performance apps is critical in today’s fast-paced digital environment to satisfy user expectations and provide businesses an advantage. The desire for developers to optimize their code and get the most out of their Node.js apps is growing with the need for scalable and efficient online applications.
Since its release in 2009, Node.js has been the most popular platform for developing server-side applications due to its event-driven, non-blocking I/O approach. But like any other technology, mastering Node.js’s design and using best practices to boost application speed is essential for obtaining optimum performance.
The article delves deep into the causes of slow Node.js applications and provides actionable advice on fixing the problem and speeding things up.
Common Challenges With NodeJs Performance
The performance of an application you build using Node.js may face its fair share of obstacles. Let’s look at a few major challenges:
Code Debt
When developers are pressed for time, they may choose quantity above quality when coding. This might cause future code to be hard to maintain or heavily reworked.
No Clear Guidelines
The adaptability of Node.js enables the creation of sophisticated programs. However, when developing with a large group, clarity and consistency in the lack of clear norms may slow progress. Setting up rules and regulations is a great way to make sure everyone is on the same page.
Inadequate Record-Keeping
Developers must have access to thorough documentation to learn the ins and outs of the Node.js framework and the individual application being developed. Lack of documentation may cause misunderstandings and delays as team members try to figure out their roles and how they relate with one another and the rest of the program.
Process Monitoring with Irregularities
Policymakers and technical officers must always keep a close eye on the application development process. If monitoring isn’t performed, problems and bottlenecks might go undetected, leading to increased expenses during development and more time spent maintaining the code after the program has gone live.
To overcome these obstacles, developers must take preventative measures, including carefully planning each step of the development process, extensively documenting the project, and keeping a close eye on the development status monitor at regular intervals.
ALSO READ : Performance measurement APIs
What are the Methods for Enhancing Node.js Performance?
The performance of your Node.js applications may be improved by implementing the following suggestions into your development workflow:
1. Use Perf Hooks
Node.js applications may benefit from using the “perf hooks” API to monitor and optimize their performance while they are being used. Here’s how this strategy can boost Node.js speed:
- Track and Evaluate Progress: With the help of perf hooks, we can annotate critical sections of code and then track how long it takes to reach those spots. Markers may be placed around your application’s most important components to help you understand how various actions and functionalities affect overall performance.
- Understand the Effectiveness of Algorithms: Using perf hooks to analyze their performance, you can determine where your algorithms may be improved. You may find performance issues in the algorithm and make informed judgments about fixing them by timing the time to execute various code branches.
- Locate malfunctions in Performance: Using perf hooks, you can track how well your program is doing while it’s being used. The acquired data might be analyzed to reveal anomalies or unexpected actions hindering productivity. This way, you may zero in on exactly which parts of your code need improvement.
- Establish attainable Goals: You may establish performance benchmarks by utilizing perf hooks to establish a baseline measure for your application’s performance. You can see how your adjustments and improvements are helping by comparing new data to your original measurements, which serve as your baseline.
- Cut Maintenance Costs: Perf hooks provide for continuous monitoring and measurement of performance, allowing for early detection of performance concerns. Taking this preventative measure lessens the likelihood of experiencing performance-related issues throughout production. By fixing these problems at the start of development, you may save money on future fixes and boost the app’s overall speed.
2. Use caching to keep the load balanced.
In cases with a big user base and heavy traffic, using caching technologies to improve the speed of Node.js apps may be quite useful. Redis and NGINX are two caching solutions that may aid with load balancing and resource optimization.
Caching prevents the program from performing resource-intensive actions on data retrieved often or is static, such as database query results or HTML files. In turn, this enhances performance and responsiveness, making for a more pleasant user experience.
3. Scale server using cluster module
The cluster module can scale Node.js applications and maximize multicore computers. Clustering improves Node.js:
- Multicore Systems: Node.js’s single-threaded ness limits it to one core. Clustering lets multicore applications use all cores. Each core may execute a child process, allowing parallel execution and task distribution.
- Load balance: Node.js server clustering distributes requests equally across child processes. This load-balancing method protects a core from overloading, improving reaction times and performance under high loads.
- Horizontal scaling: Clustering horizontally scales Node.js on a single machine. Adding cores to your system lets you grow the program by establishing additional child processes. This scalability helps the program manage additional traffic and queries.
- High Availability: Clustering lets the controller process continue a child process that crashes or stops responding. This avoids downtime by swiftly recovering from errors without harming the application.
- Increased ROI: Clustering improves hardware usage without adding servers or infrastructure. You can manage growing traffic and user demand without major infrastructure investments, which boosts ROI.
4. Application’s asynchronous mode
While Node.js is well-known for its non-blocking, single-threaded asynchronous functions, it is important to be wary of any synchronous code that may have made its way into your application. There is a performance hit and a phenomenon called “Callback Hell” when synchronous code blocks the event loop.
Execution of synchronous code might delay the main thread, degrading the web application’s performance as a whole. Functions may have synchronized code introduced by modules or external libraries that use synchronous operations.
To improve Node.js’ performance, programmers should avoid using synchronous code by accident instead of asynchronous approaches wherever feasible. Node.js’s asynchronous nature is a boon to performance, and you can keep it that way by adopting non-blocking procedures that allow for continuous application processing.
5. Accelerate data retrieval by optimizing queries.
To efficiently get information from huge databases, query optimization is essential. Node.js applications may enhance the user experience by optimizing query functions by making data retrieval and display quicker. The application’s query optimizations mean less memory is used during processing. This method improves efficiency and helps reduce the expenses businesses incur from constantly updating their data and rewriting their code. Node.js applications may deliver smooth and quick data retrieval by simplifying the querying process, improving overall performance and user happiness.
6. Combining WebAssembly and Rust in Node.js
Adding WebAssembly and Rust to Node.js improves efficiency and processing speed by using the advantages of both languages in conjunction with one another. This novel technique, developed by Josh Hannaford, combines the portability and speed of WebAssembly with the efficiency and safety of Rust. Faster execution and reduced binary sizes result from combining Rust with WebAssembly during compilation, which is used by Node.js applications.
Regarding speed, this method is superior to similar implementations in Java, Go, and Python. This compilation approach provides portability, flexibility, and higher developer productivity in response to the ever-changing digital market, allowing firms to fulfill stringent standards and produce results more easily and expeditiously.
7.Avoid Memory Leaks
Memory leaks must be avoided at all costs to keep Node.js applications running smoothly and quickly. Over time, memory use rises due to leaks, which occur when memory is allocated but not properly released. Developers need to be aware of event listeners and closures and ensure the correct handling of variables to prevent memory leaks. Memory leaks may cause performance issues and crashes. Therefore, monitoring how much memory is being utilized is important and undertaking extensive testing regularly to find and fix them is important.
8. Use Worker Threads for CPU-Intensive Tasks
Node.js applications can handle CPU-heavy tasks more efficiently by using worker threads. These threads allow you to perform tasks without blocking the main event loop.
Worker threads run separately from the main thread and other workers. They can share data by passing `ArrayBuffer` or `SharedArrayBuffer` instances. Additionally, they communicate with the main thread via a message channel, allowing data to be sent back and forth between them.
9. Scalable Client-Side Authentication with JWT
Many web applications need to track user sessions to create a personalized experience. Traditionally, stateful authentication is used, where a session ID is generated and stored on the server, often in a centralized storage system like Redis. However, this approach can be limiting, especially when scaling multiple servers.
A more scalable solution is stateless authentication using JSON Web Tokens (JWT). With JWT, a token containing user information in a base64-encoded format is generated when a user logs in. This token is then used for all subsequent API requests, allowing the server to authenticate the user without storing session data.
By using JWT, you avoid the complications of managing state across multiple servers, making it easier to scale your application. A Node.js development company can help you implement JWT effectively, ensuring your application remains secure and scalable.
10. Enhance WebSockets for Server Communication
In a traditional HTTP request/response model, each request requires setting up a new connection between the client and server. This can add overhead and slow communication, especially in real-time applications like chat or gaming.
WebSockets offer a solution by maintaining a persistent connection between the client and server. This reduces the need for repeatedly setting up and tearing down connections, leading to faster and more efficient communication.
In Node.js applications, where handling many concurrent connections is common, using WebSockets can significantly improve performance by reducing the overhead associated with managing short-lived connections.
11. Improve Performance with HTTP/2 and SSL
HTTP/2 introduces two key features that enhance web application performance:
a. Header Compression: HTTP/2 compresses HTTP headers, reducing the size of data being transmitted and making the process more efficient.
b. Multiplexing: This feature allows multiple requests and responses to be sent simultaneously over a single TCP connection, minimizing the time and resources needed to establish connections.
To use HTTP/2 with Node.js, you must implement TLS (Transport Layer Security) and SSL (Secure Socket Layer) protocols. Fortunately, Node.js makes it easy to set up an HTTP/2 server.
Final Thoughts
Optimizing Node.js application performance is essential for ensuring a smooth user experience. Developers should watch out for common issues like blocking the event loop, using inefficient algorithms and making too many database queries. By following best practices and using performance-focused frameworks, you can improve your Node.js applications.
Need professional support to optimize your node.js app performance? Hire Node.js developers from Valuetree who specialize in optimization and scaling for larger or more complex projects. We can help you enhance your application’s performance and responsiveness.
FAQs
How can I gauge how well my Node.js app is performing?
Node.js’s perf hooks API allows you to tag and monitor your app’s performance while it’s being used. Time spent in execution may be monitored to fix slow spots in the code.
For what reasons is Node.js clustering so crucial?
When running on a multicore machine, Node.js applications may use more than one CPU core, thanks to clustering. It offers horizontal scalability, load balancing, and high availability, guaranteeing reliable service even under heavy use.
What are the common performance pitfalls in Node.js applications?
Some common performance issues in Node.js applications include blocking the event loop, using inefficient algorithms, and making too many database queries. These problems can slow down your application and result in slower response times.
Which NodeJS frameworks or libraries do you use for high-performance applications?
Several Node.js frameworks and libraries, including Satisfy, Koa, and Expressjs, are designed to boost performance. Fastify is known for its speed and low overhead, making it ideal for building fast APIs. Koa, on the other hand, focuses on simplicity and modularity while still delivering strong performance.
Contact Valuetree to enhance your Node.js application’s performance and scalability!